Introduction
The dyncall library encapsulates architecture-, OS- and compiler-specific
function call semantics in a virtual bind argument
parameters from left to right and then call interface allowing programmers
to call C functions in a completely dynamic manner. In other words, instead of
calling a function directly, the dyncall library provides a mechanism to
push the function parameters manually and to issue the call afterwards.
This means, that a program can determine at runtime what function to call, and
what parameters to pass to it. The library is written in C and assembly and
provides a very simple C interface to program against.
The library comes in very handy to power flexible message systems, dynamic
function call dispatch mechanisms, closure implementations, to bridge
different programming languages, or to simply wrap a "vararg"
function.
When it comes to language bindings, the dyncall library provides a clean
and portable C interface to dynamically issue calls to foreign code using
small call kernels written in assembly. Instead of providing code for every
bridged function call, which unnecessarily results in code bloat, only a
couple of instructions are used to invoke every possible call.
Example
Let's say, we want to make a call to the function:
Using the dyncall library, this function would be called as follows:
DCCallVM* vm = dcNewCallVM(4096);
dcMode(vm, DC_CALL_C_DEFAULT);
dcReset(vm);
dcArgDouble(vm, 4.2373);
r = dcCallDouble(vm, (DCpointer)&sqrt);
dcFree(vm);
If you already have a DCCallVM object (e.g. for followup calls), this simply boils down to:
dcArgDouble(vm, 5.2); // Push/bind argument(s).
r = dcCallDouble(vm, (DCpointer)&sqrt); // Call.
Note that, by exposing this functionality to a dynamic scripting environment, the latter can gain system programming status to a certain degree. It is easy to see the power one can get by calling a C function directly from within a scripting language. Demonstrative bindings and examples for several different scripting languages are provided with the library.
Rough overview of platforms and features
The dyncall library runs on many different platforms and operating systems (including Windows, Linux, OpenBSD, FreeBSD, macOS, DragonFlyBSD, NetBSD, Plan9, iOS, Haiku, Nintendo DS, Playstation Portable, OpenSolaris, Minix, Raspberry Pi, ReactOS, etc.) and processors (x86, x64, arm (arm & thumb mode), arm64, mips, mips64, ppc32, ppc64, sparc, sparc64, etc.).
Most of the platforms' C-calling conventions are supported - including
"vararg" functions, as well es C++-"thiscalls" (on some
platforms), syscalls (on some platforms), and the multitude of calling
conventions on Windows ("fastcall", "stdcall", etc.) or some
embedded platforms (e.g. atpcs, eabi, apple, armhf on arm, ...).
Most of C99's types are supported for setting up a call, however, support for
passing structures and unions by value is only partially implemented (currently
only for x64 platforms).
Additionally, dyncall comes with dyncallback, a library for callback support, and dynload, to facilitate portable dynamic library symbol loading and access (only for platforms with dynamic library support).
The feature matrix below gives a brief overview of the currently supported
platforms (as of the repo's tip). Different colors are used, where a
green
cell indicates a supported
platform, with both, call and callback support, yellow
a platform that might work (but is untested) and red
a platform that is currently unsupported. Gray cells are combinations that don't
exist at the time of writing, or that are not taken into account.
Light green
cells mark complete feature support, including passing aggregates
(struct, union) by value. Dark green
means basic support but lacking lesser used features (e.g. no aggregate-by-value
support). Please note that a green cell (even a light-green one) doesn't imply
that all existing calling conventions/features/build tools are supported for that
platform (but the most important).
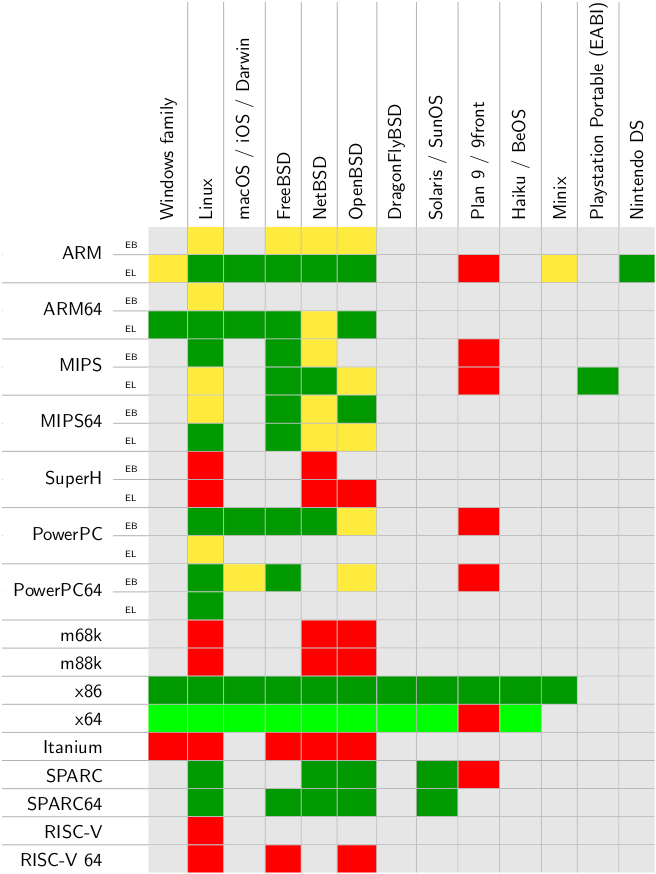
For details, refer to the dyncall manual/documentation.
For information about how we test and build a majority of the supported platforms, check out dynOS.